There are reasons to prefer developing Bluetooth applicationsin C instead of in a high level language such as Python. The Pythonenvironment might not be available or might not fit on the target device;strict application requirements on program size, speed, and memory usage maypreclude the use of an interpreted language like Python; the programmer maydesire finer control over the local Bluetooth adapter than PyBluez provides;or the project may be to create a shared library for other applications tolink against instead of a standalone application.As of this writing, BlueZ is a powerful Bluetooth communications stack withextensive APIs that allows a user to fully exploit all local Bluetoothresources, but it has no official documentation. Furthermore, there is verylittle unofficial documentation as well. Novice developers requestingdocumentation on the official mailing lists
This chapter presents a short introduction to developing Bluetoothapplications in C with BlueZ. The tasks covered in chapter 2 are nowexplained in greater detail here for C programmers.
Note: Replace the C: RepairSource Windows placeholder with the location of your repair source. For more information about using the DISM tool to repair Windows, reference Repair a Windows Image. At the command prompt, type the following command, and then press ENTER. C is a compiled language meaning your program's source code must be translated (compiled) before it can be run on your computer. VS Code is first and foremost an editor, and relies on command-line tools to do much of the development workflow. The C/C extension does not include a C compiler or debugger. Hone your C programming skills with the UVa Online Judge. If you are using Windows, you can use the MinGW Windows tutorial to help prepare programs. This website has thousands of datasheets, and is maintained by an engineer that has spent 3 years collecting datasheets as a service to the engineering community. Initially, the address of c is assigned to the pc pointer using pc = &c. Since c is 5,.pc gives us 5. Then, the address of d is assigned to the pc pointer using pc = &d. Since d is -15,.pc gives us -15.
A simple program that detects nearby Bluetooth devices is shown in Example 4-1. The program reserves system Bluetoothresources, scans for nearby Bluetooth devices, and then looks up the userfriendly name for each detected device. A more detailed explanation of thedata structures and functions used follows.
Example 4-1. simplescan.c
4.1.1. CompilationTo compile our program, invoke
The basic data structure used to specify a Bluetooth device address is the


Local Bluetooth adapters are assigned identifying numbers starting with 0, anda program must specify which adapter to use when allocating system resources.Usually, there is only one adapter or it doesn't matter which one is used, sopassing
It is
If there are multiple Bluetooth adapters present, then to choose the adapterwith address ``01:23:45:67:89:AB', pass the
Most Bluetooth operations require the use of an open socket.
After choosing the local Bluetooth adapter to use and allocating systemresources, the program is ready to scan for nearby Bluetooth devices. In theexample,
If
The
For the most part, only the first entry - the
Once a list of nearby Bluetooth devices and their addresses has been found,the program determines the user-friendly names associated with thoseaddresses and presents them to the user. The

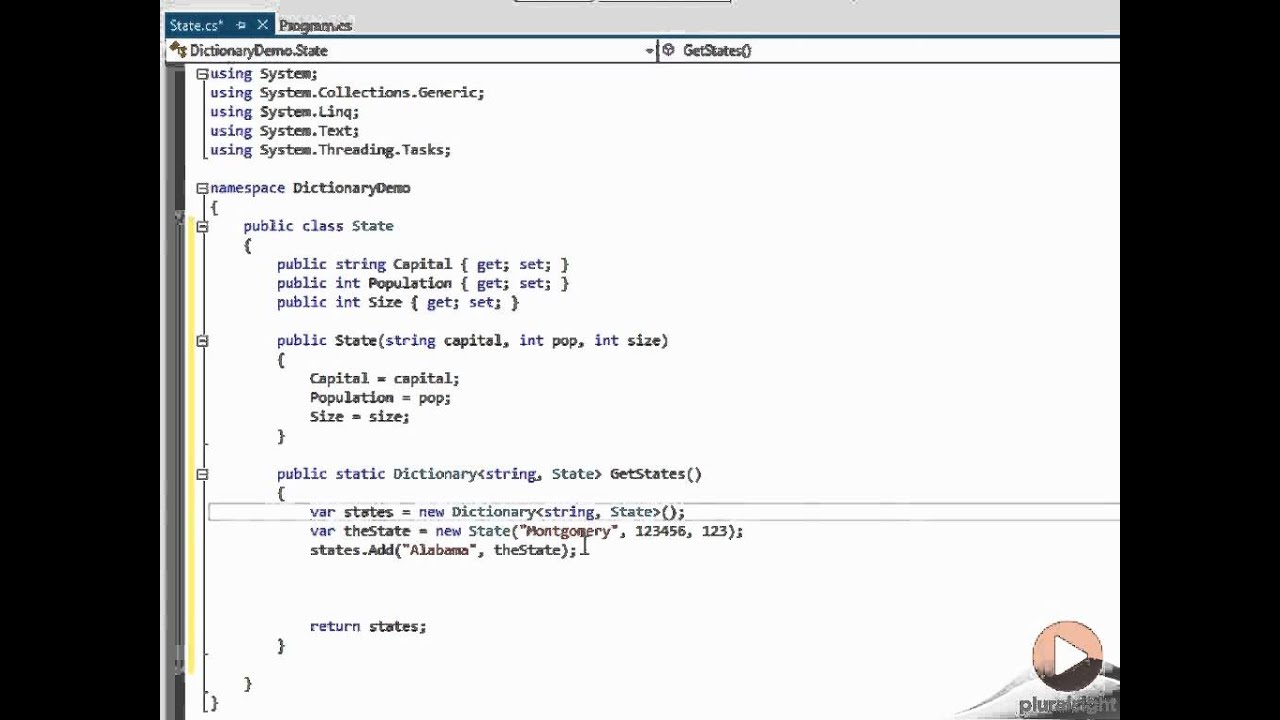
http://www.bluez.org/lists.html Game genie codes for snes9x emulators.
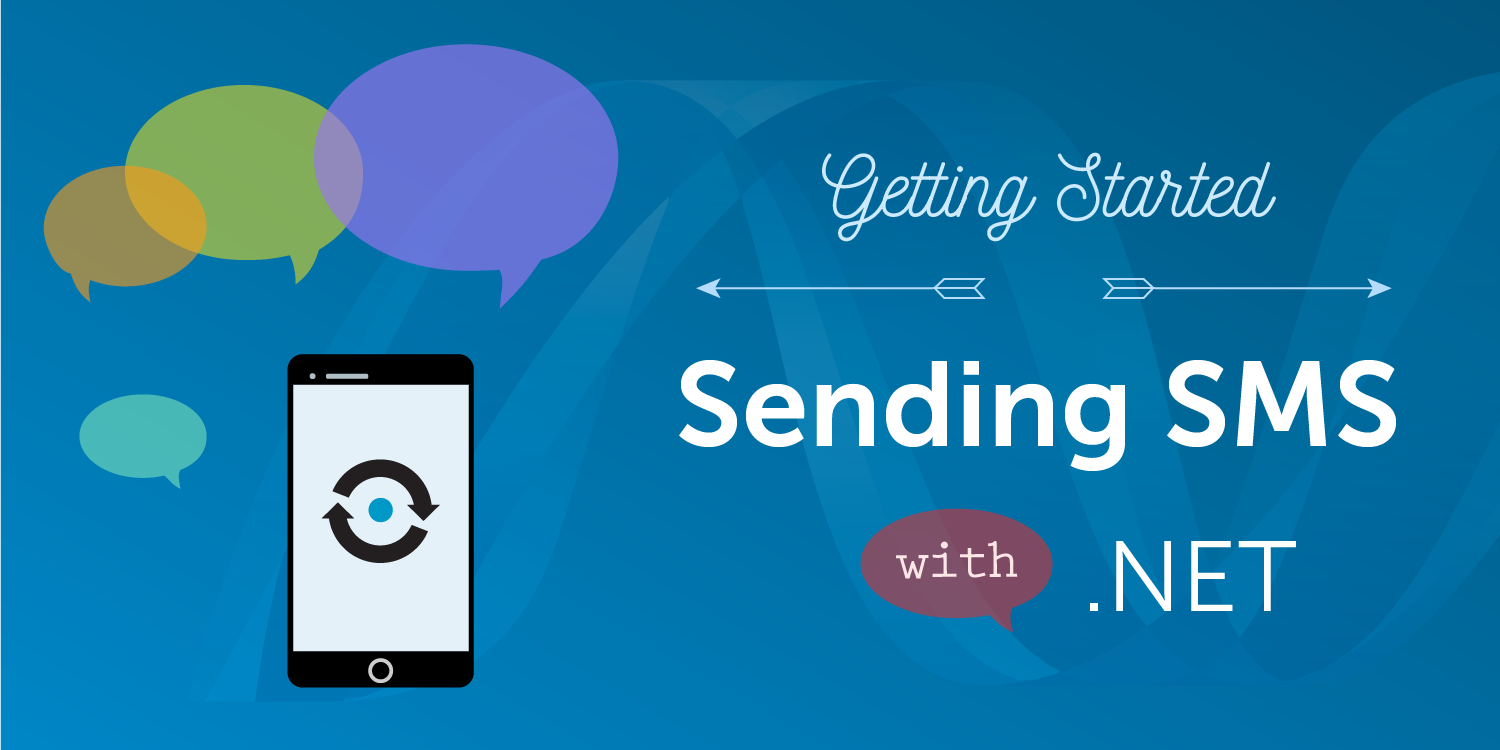
for thecurious, it makes a call to
https://www.bluetooth.org/foundry/assignnumb/document/baseband
[4]http://www.bluetooth.org/spec
About C Programming
- Procedural Language - Instructions in a C program are executed step by step.
- Portable - You can move C programs from one platform to another, and run it without any or minimal changes.
- Speed - C programming is faster than most programming languages like Java, Python, etc.
- General Purpose - C programming can be used to develop operating systems, embedded systems, databases, and so on.
Why Learn C Programming?
- C helps you to understand the internal architecture of a computer, how computer stores and retrieves information.
- After learning C, it will be much easier to learn other programming languages like Java, Python, etc.
- Opportunity to work on open source projects. Some of the largest open-source projects such as Linux kernel, Python interpreter, SQLite database, etc. are written in C programming.
How to learn C Programming?
Using C# In Visual Studio Code
- C tutorial from Programiz - We provide step by step C tutorials, examples, and references. Get started with C.
- Official C documentation - Might be hard to follow and understand for beginners. Visit official C Programming documentation.
- Write a lot of C programming code - The only way you can learn programming is by writing a lot of code.